If you are connecting the sensor to a Analog Protoboard Adapter wired to Arduino pin A2, you will need to build a voltage-divider circuit on a breadboard with two resistors and a diode to change the sensor output from +/-10 volts to 0 to 5 volts.Â
A simple circuit is shown here.
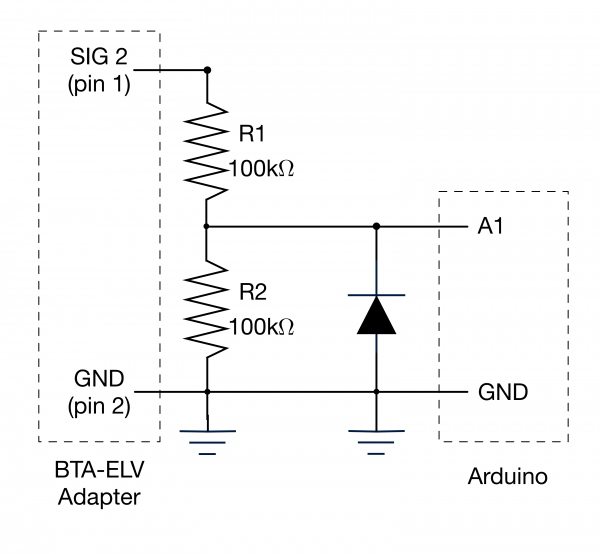
Simplified Circuit for Use with +/-10 Volt Sensors